Updated January 9, 2020
Avoid React Native Splash Screen Flash on iOS (the easy way)
When first opening your React Native app you may noticed a flash between the splash screen and the app starting. Historically, I've gone through a complex/confusing process that was often error prone to fix this.
Thanks to stumbling across a few lines in the docs I realized this problem is really easy to fix on iOS!
Here's the problem.
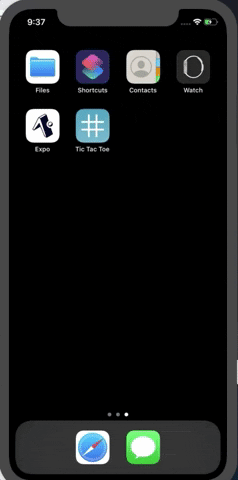
It's very subtle, especially as a gif, but you can see a brief flash. As your app gets more complex this may get more exaggerated. Fortunately, the fix is easy.
Open AppDelegate.m
, find the didFinishLaunchingWithOptions
method, and paste the following between [self.window makeKeyAndVisible];
and return YES;
UIView* launchScreenView = [[[NSBundle mainBundle] loadNibNamed:@"LaunchScreen" owner:self options:nil] objectAtIndex:0];
launchScreenView.frame = self.window.bounds;
rootView.loadingView = launchScreenView;
This will leave you with a didFinishLaunchingWithOptions
method similar to this:
AppDelegate.m
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
RCTBridge *bridge = [[RCTBridge alloc] initWithDelegate:self launchOptions:launchOptions];
RCTRootView *rootView = [[RCTRootView alloc] initWithBridge:bridge
moduleName:@"TicTacToeInit"
initialProperties:nil];
rootView.backgroundColor = [[UIColor alloc] initWithRed:1.0f green:1.0f blue:1.0f alpha:1];
self.window = [[UIWindow alloc] initWithFrame:[UIScreen mainScreen].bounds];
UIViewController *rootViewController = [UIViewController new];
rootViewController.view = rootView;
self.window.rootViewController = rootViewController;
[self.window makeKeyAndVisible];
UIView* launchScreenView = [[[NSBundle mainBundle] loadNibNamed:@"LaunchScreen" owner:self options:nil] objectAtIndex:0];
launchScreenView.frame = self.window.bounds;
rootView.loadingView = launchScreenView;
return YES;
}
That's it!
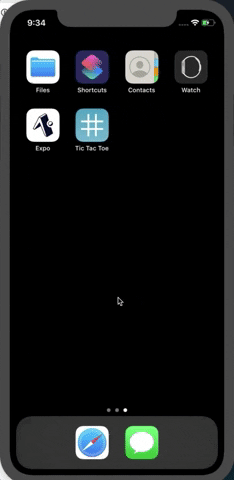