Updated August 17, 2016
Preparing a React Native Android App for Production
Originally publish on medium.com.
We’re currently going through the launch process of one of our client’s apps — an app built with React Native running on both iOS and Android.
Using React Native with Android is easy, it just works. Until you have to push that app into the app store. Then you’re hit with terms like signing your APK, .keystore files, and so on. If you’re anything like me you’ll be confused, intimated, and you’re going to make mistakes. That’s okay. Let’s make the mistakes when there is a low/no cost involved.
Before continuing I have to say that this is basically a rehash of the official documentation on “Generating a Signed APK”.
Terms
First thing I want to do is define a few terms. Hopefully to help you understand them, mostly to make sure I have a grasp on them.
APK File
Much like on the Window’s .exe or iOS’s .ipa — .apk is simply the Android file format that it understands how to execute. There’s a lot more to it but we’re keeping things simple.
Keystore File
This is the one that really confused be because I hadn’t used it when building iOS apps with React Native. I’m not sure if this is right but I think about a keystore as a certificate. It’s really important and it identifies that you (or anyone you share it with) is authorized to publish a new version of that app. Make sure you keep this secure and back it up.
Gradle
Gradle is a build tool that Android uses. Its’ going to do all of the heavy lifting for us behind the scenes. I’m very grateful for it because it keeps things relatively simple for me.
Okay, enough of the terms. Let’s get to actually prepping our app. Obviously the first thing we’ll do is create our React Native app.
react-native init AwesomeProject && cd AwesomeProject
So we’ve got a fully functioning app that runs on Android (and iOS) now!
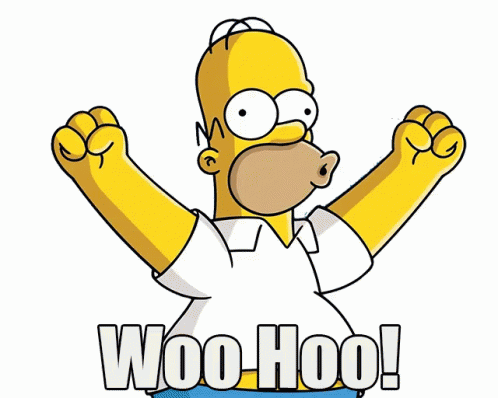
When things work after writing one line
First thing we’ll want to do now is generate our keystore file. We’ll want to run the following command. Note: You’ll want to change my-release-key.keystore and my-key-alias to something that makes sense for you.
keytool -genkey -v -keystore my-release-key.keystore -alias my-key-alias -keyalg RSA -keysize 2048 -validity 10000
It’s then going to ask you for a password and to confirm the password (remember the password).
Once you get through that you’ll be asked for some identifying information to add to the keystore. You’ll probably want to add real data.
Finally you’ll be prompted to set and confirm another password (make sure to remember this one as well).
And there you have it! You’ve now got a production keystore file you can use to publish your Android app! Back up this file, remember the passwords, and share it with whomever has the ability to publish your app.
Wait, what?
Okay, maybe that was a little fast. Let’s run through that in a little more detail.
First we’re generating a keystore file with keytool, setting the name of the file, setting an alias, and making this key valid for 10,000 days.
The first password prompt is asking you to set a password for the keystore itself. You’ll want to remember this password. It’s then going to ask you for your organizational information. Add whatever is appropriate for you. This is stored within the keystore file.
Finally you’re asked for another password. This one is associated with your alias. Remember this password as well and remember that it’s for the alias, not the key store.
Adding the Keystore to the App
Depending on where you created the file you’ll want to, after backing it up (please remember to back this up and never lose it), you’ll want to put a copy of the file in AwesomeProject/android/app.
You’re probably going to want to update your .gitignore as well to make sure you don’t check it into source control. I’m not sure if this is necessary but when it comes to keys/certificates I like to play it safe.
Once that’s done you’ll want to make sure gradle has all the necessary info. This is going to happen in ~/.gradle/gradle.properties which is NOT the gradle.properties inside of your project. It’s a global file that’s accessed whenever gradle is used. We’ll add a few variables in there, make sure to scope them to your project because you could have multiple values in there representing the same thing. Use the file name, alias, and password you set up earlier.
MY_AWESOME_PROJECT_RELEASE_STORE_FILE=my-release-key.keystore
MY_AWESOME_PROJECT_RELEASE_KEY_ALIAS=my-key-alias
MY_AWESOME_PROJECT_RELEASE_STORE_PASSWORD=keystore-password
MY_AWESOME_PROJECT_RELEASE_KEY_PASSWORD=keyalias-password
Boom, set up. Next, and finally, we’ll inform our app about which variables to access from the gradle properties. This is going to take place in android/app/build.gradle.
You’ll have to scroll down towards the bottom of the file inside the android block. I’m going to add this just below defaultConfig.
// Removed for brevity
defaultConfig {
applicationId "com.awesomeproject"
minSdkVersion 16
targetSdkVersion 22
versionCode 1
versionName "1.0"
ndk {
abiFilters "armeabi-v7a", "x86"
}
}
signingConfigs {
release {
storeFile file(MY_AWESOME_PROJECT_RELEASE_STORE_FILE)
storePassword MY_AWESOME_PROJECT_RELEASE_STORE_PASSWORD
keyAlias MY_AWESOME_PROJECT_RELEASE_KEY_ALIAS
keyPassword MY_AWESOME_PROJECT_RELEASE_KEY_PASSWORD
}
}
buildTypes {
release {
minifyEnabled enableProguardInReleaseBuilds
proguardFiles getDefaultProguardFile("proguard-android.txt"), "proguard-rules.pro"
signingConfig signingConfigs.release // add this line as well
}
}
// Removed for brevity
Fin
Finally you’ll want to generate your production apk (app-release.apk) with the following commands:
cd android && ./gradlew assembleRelease
Hey, you’re done! It’s not too scary. This may be simple but I wanted to make sure I had a grasp and wanted to add a little bit more to the default docs (apologies if I used too many words).
The final apk that you would upload to the Play Store is in AwesomeProject/android/app/build/outputs/apk/.
Bonus: Testing the Build
If you want to give this a shot and make sure everything is running go ahead and run
cd android && ./gradlew installRelease
This will jump into your android directory and actually install the real build on your device. Make sure you’ve got either a device connected or an emulator running. The installation that is a result of installRelease will only work if you’ve done the above steps correctly so if it runs you’re good to go!