Updated August 29, 2017
React Native Basics: Copy to Clipboard
Originally publish on medium.com.
Reading and writing from the clipboard is incredibly easy in React Native by using the Clipboard API. In this quick tutorial we’ll build a simple app to read and write to it.
Starting App
To keep this tutorial simple we’ll start with a very basic app. It’s got two buttons, a TextInput, and some areas to render text.
App.js
import React from 'react';
import { StyleSheet, Text, View, Button, TextInput } from 'react-native';
export default class App extends React.Component {
constructor(props) {
super(props);
this.state = {
text: '',
clipboardContent: null,
};
}
readFromClipboard = () => {
alert('TODO: Read from Clipboard');
};
writeToClipboard = () => {
alert('TODO: Write to Clipboard');
};
render() {
return (
<View style={styles.container}>
<Text style={styles.boldText}>Clipboard Contents: </Text>
<Text>{this.state.clipboardContent}</Text>
<Button onPress={this.readFromClipboard} title="Read from Clipboard" />
<View style={styles.seperator} />
<TextInput
style={styles.textInput}
onChangeText={(text) => this.setState({ text })}
value={this.state.text}
placeholder="Type here..."
/>
<Button onPress={this.writeToClipboard} title="Write to Clipboard" />
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#fff',
alignItems: 'center',
justifyContent: 'center',
},
boldText: {
fontWeight: '600',
},
seperator: {
height: StyleSheet.hairlineWidth,
backgroundColor: 'gray',
width: '80%',
marginVertical: 40,
},
textInput: {
height: 40,
borderColor: 'gray',
borderWidth: 1,
width: '80%',
paddingHorizontal: 10,
},
});
Reading from the Clipboard
Let’s say you want to copy a password from the user’s clipboard — unless that text is going into an input the user can’t press and hold to copy it so we want to give them a simple button to copy it instead.
That’s where Clipboard.getString()
comes in. This function returns a promise with either the value or an error.
First, make sure you import { Clipboard } from 'react-native';
.
You can handle promises a few different ways but I’ll be opting to use async/await because it keeps are code very succinct.
Everything is already wired up (the button press will call the readFromClipboard
function) so we can just focus on interacting with the Clipboard API.
Since we’re using async/await we need to put async
in front of our function.
readFromClipboard = async () => {
alert('TODO: Read from Clipboard');
};
Then inside that function we’ll get the clipboard value and save it to state. Since getString
is a promise we need to wait for that value, which we can do by using the await
keyword.
readFromClipboard = async () => {
const clipboardContent = await Clipboard.getString();
this.setState({ clipboardContent });
};
That will then display the contents of the keyboard below “Clipboard Contents:”!
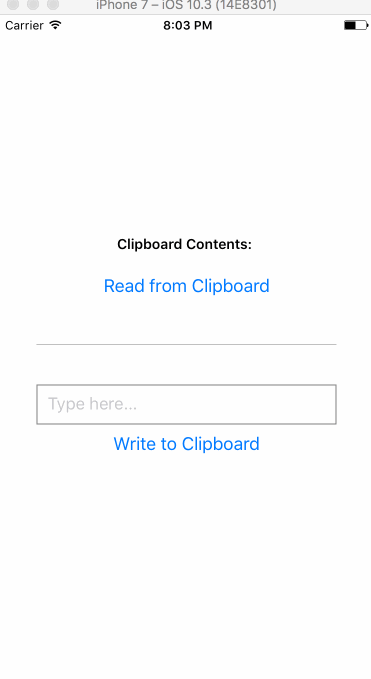
Try copying a contact’s name and the pasting it in the app.
Writing to the Clipboard
Writing to the clipboard is almost exactly the same so I’ll keep this one more brief. Once we successfully write to the clipboard we’ll alert that we did so to the user.
Note: The user could just as easily copy the value directly from the input, but where’s the fun in that?
First, make the function work with the await
keyboard by putting async
in front of it.
writeToClipboard = async () => {
alert('TODO: Write to the Clipboard');
};
We then want to write the value in our input to the clipboard and alert the user once it’s completed.
writeToClipboard = async () => {
await Clipboard.setString(this.state.text);
alert('Copied to Clipboard!');
};
Go ahead and copy something from your app, paste it into another app, or copy it to the clipboard and then read it from the code we wrote in the previous section!
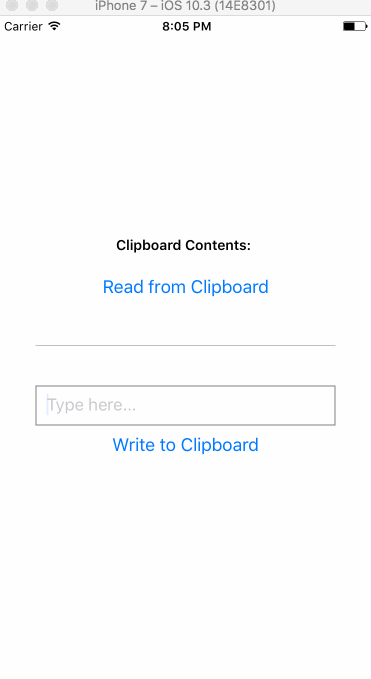
Writing data from clipboard
And there we go! It’s a very simple API to work with but sometimes an example helps.